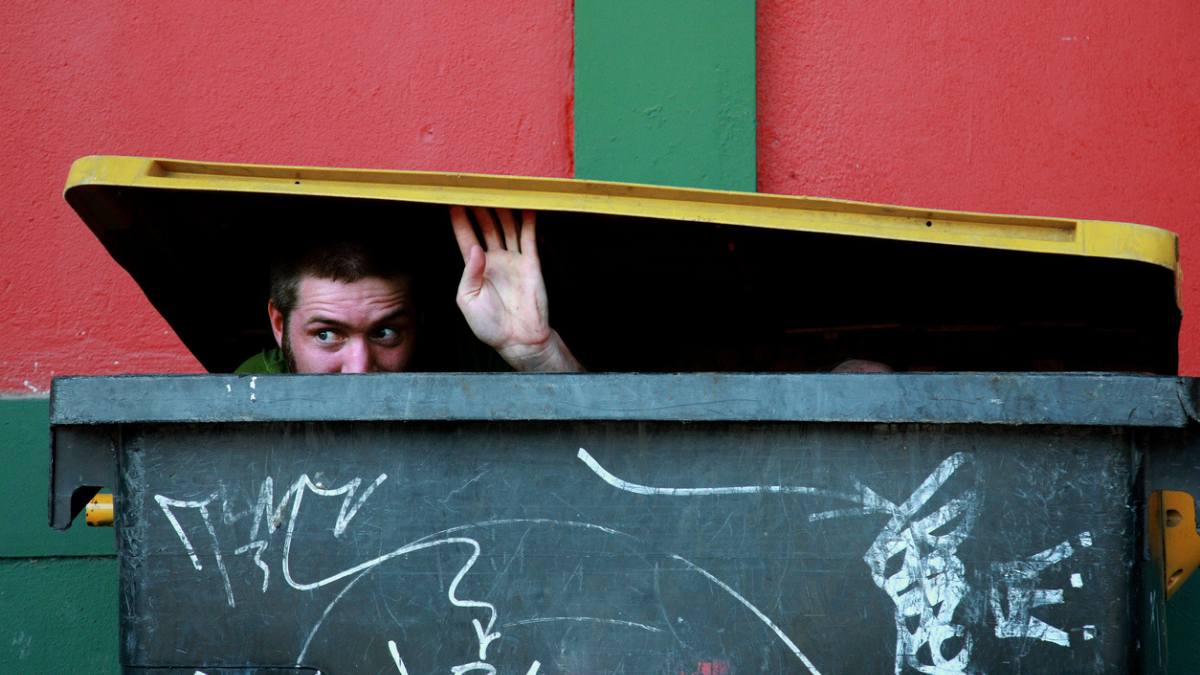
Before going to how GC works in Android, we need to understand how memory allocation works in Android
public class HomeActivity extends AppCompatActivity {
private MyObject myObject;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
myObject = new MyObject();
}
}

If we look at memory allocation for the above piece of code then it looks like👇
- HomeActivity instance is created.
- When onCreate() method is called, it instantiate MyObject() object.
- At this moment, we have two objects residing into the memory.
- And then the instance of MyObject() is assigned to internal private field of HomeActivity.
- So HomeActivity now has a reference of the MyObject() and can reach it whenever need it.
So whenever new objects are created, they consume some amount of available memory.
If allocated memory never releases, sooner or later the application will crash with OutOfMemoryException.
Garbage Collector (GC) is a system process that automatically reclaims memory by discarding objects that are no longer in use.
And this description raises another question…and thats
HOW DOES GC KNOWS WHICH OBJECTS ARE NO LONGER IN USE…?
To understand how the garbage collector works, you need to understand the concept of object reachability.
Object Reachability
Please follow the diagram whenever we look for some specific object in the memory is reachable or not.

So if you perform this flow for each allocated object in the memory you will understand which of them are reachable and which of them are not.
Important
Whenever the object is determined to be not reachable then it’s considered to be not in use and then it is eligible for garbage collection. So basically GC will automatically determine which objects in memory are not reachable anymore and then it will release the memory associated with these objects.
Example

- is :MyObject2 is reachable ?
- is :MyObject2 a root ? — NO
- is :MyObject2 referenced by any other object ? — YES
- is :MyObject1 a root ? — NO
- is :MyObject1 referenced by any other object ? — YES
- is :HomeActivity is reachable ?
Note: What is Root – will see in later part in this blog.
:MyObject1 and :MyObject2 are reachable if :HomeActivity is reachable (Transitivity)
Under normal condition, Activity objects are reachable until after Android invokes their onDestroy() callback.
Special Edge case : Circular Reference

Here two objects are pointing to one another if the GC algorithm is totally naive it will get into an infinite loop between these two objects, but GC is not that stupid, in this specific case if there are no other references to either of the objects, both will be considered as unreachable and will be eligible for GC.
GC can handle circular references involving many more objects. So regardless of how many objects are there in the circle of chain, GC will understand that these objects basically point to one another and if there are no external references to that group of objects, all of them will be considered non-reachable and their memory will be reclaimed.
Thumb Rule
All object in your application must be garbage collected eventually** except for objects that you explicitly want to use for the entire life of application.
Bonus Topics 😉😉
Root
These are the objects which are always considered reachable, thus never cleaned by GC.
Roots are the objects which hold other object’s memory.

Important Roots in Android Application
- Object referenced from static fields
- Custom Subclass of Application class
- Live Threads — Click Here
Memory Leaks
Object that is not garbage collected after it’s no longer used inside the application.
To read more on Memory Leaks Click Here
I hope you enjoyed reading this article. Please let me know your suggestions and comments.
If you have any questions or comments, please join the forum discussion below!
Stay connected on LinkedIn Twitter and StackOverFlow