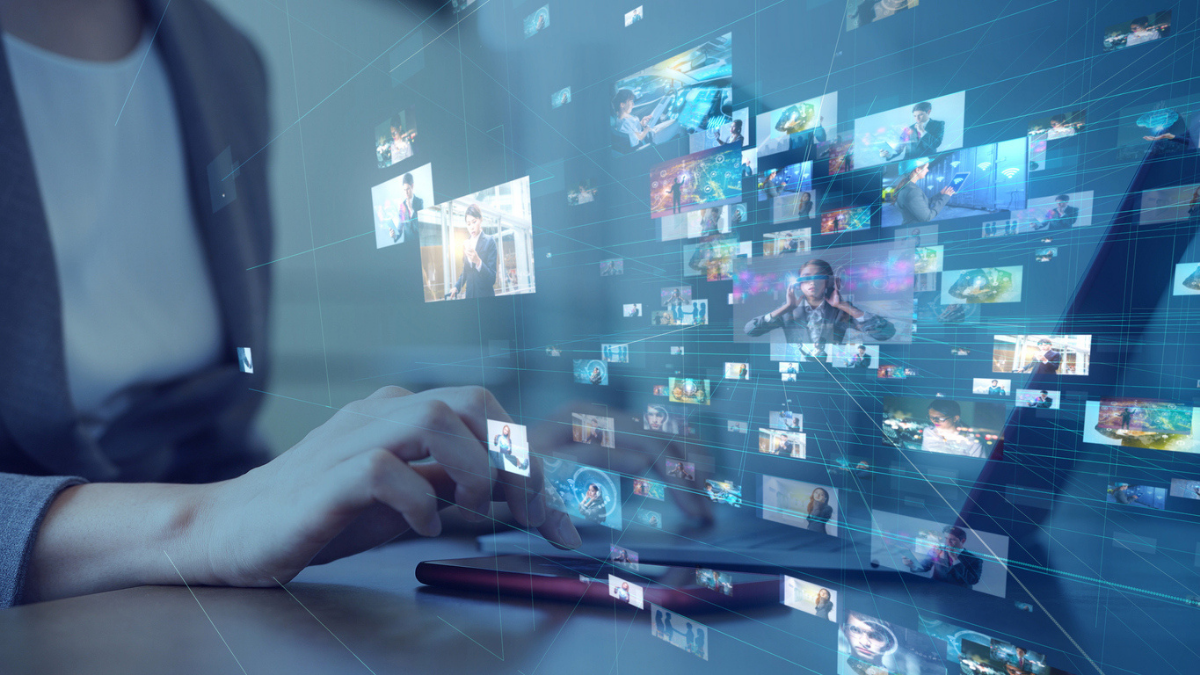
A lot of android apps require the use of images and thus the need for images to be loaded efficiently and quickly arises. Not only that but we also need to handle caching so that images are loaded quicker while also ensuring that the correct size of the image is being loaded in the memory.
To make such tasks easier we use image loading libraries. But as there is an abundance of such libraries it’s hard to choose which one to use for your project. Well don’t worry, to make things simpler for you we will discuss the most popular ones in this article, so let’s start!
Glide
So the official documentation for Glide describes it as :
A fast and efficient image loading library for Android focused on smooth scrolling.
To use Glide in your app you need to add the following lines to your app-level build.gradle :

And the following one to your AndroidManifest File :

That’s it, you can now use Glide to load images from URL or drawable images. The Glide API is quite simple to use, here’s an example :

Transformations
Glide includes a lot of transformations like :
- CenterCrop
- FitCenter
- CircleCrop
Here’s an example of how a transformation is used is in Glide :

Advantages
- Supports GIF Animation
- Simple to use
- High caching speed
Disadvantages
- Big library size
Picasso
Picasso is an image loading library developed by Square. The documentation describes it as :
A powerful image downloading and caching library for Android
To use Picasso in your app, you need to add the following lines to your app-level build.gradle :

You also need to add the Internet Permission as with Glide.

Picasso’s API is really simple to use as well, here’s an example :
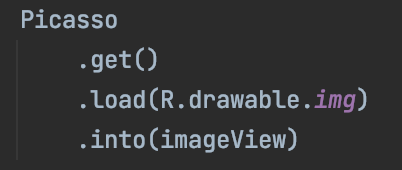
Transformation
Picasso supports a lot of transformations as well, you can use them like this :

Advantages
- Simple to use
- Small download size
Disadvantages
- No GIF support
Fresco
Fresco is an image loading library developed by Facebook, on its official website it’s described as :
Fresco is a powerful system for displaying Android applications. It takes care of image loading and display so you don’t have to.
Add the following lines to your build.gradle file :

Add the internet permission in your AndroidManifest :

Initialize Fresco in your MainActivity class :

To use it in your app create a SimpleDraweeView in xml file :
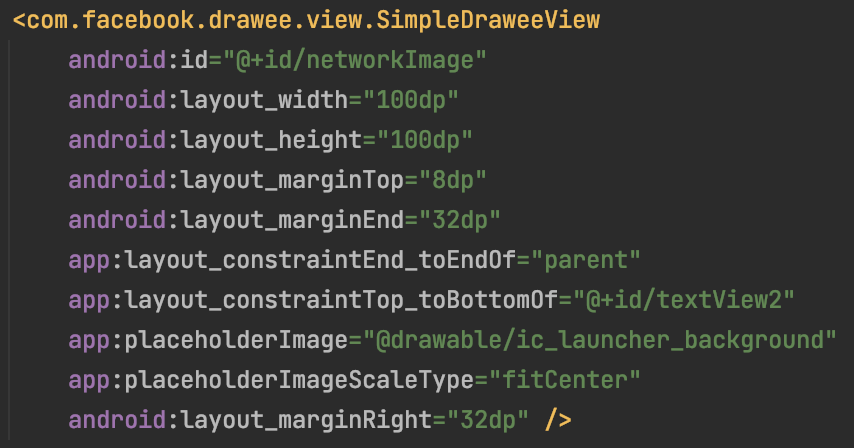
And use the Fresco API to set an image to it :

Transformations
Applying transformations in code is a bit more complex in Fresco than in Glide and Picasso which are quite similar in their approach. However, most transformations can be easily applied in the XML layout, for example :

Advantages
- Supports GIF Animation
- Streaming Functionality
Disadvantages
- Big Library Size
- SimpleDraweeView does not support wrap content
COIL
In its documentation, the coil is described as :
An image loading library for Android backed by Kotlin Coroutines.
To use COIL in your app, add the following to build.gradle

Add the internet permission as usual :

Use the API as following :

Transformations
COIL provides four transformations by default :
- BLUR
- Circle Crop
- Gray Scale
- Rounded Corners
Here’s an example of how to use transformations in COIL :

Advantages
- Simple to use
- Made for kotlin
Disadvantages
- Not fully compatible with Java
Comparison
Finally, here’s a comparison chart comparing all four :

Conclusion
In conclusion, I would say that COIL is good for small projects as it is super easy to use while Glide would work better for large projects with its robust cache system.