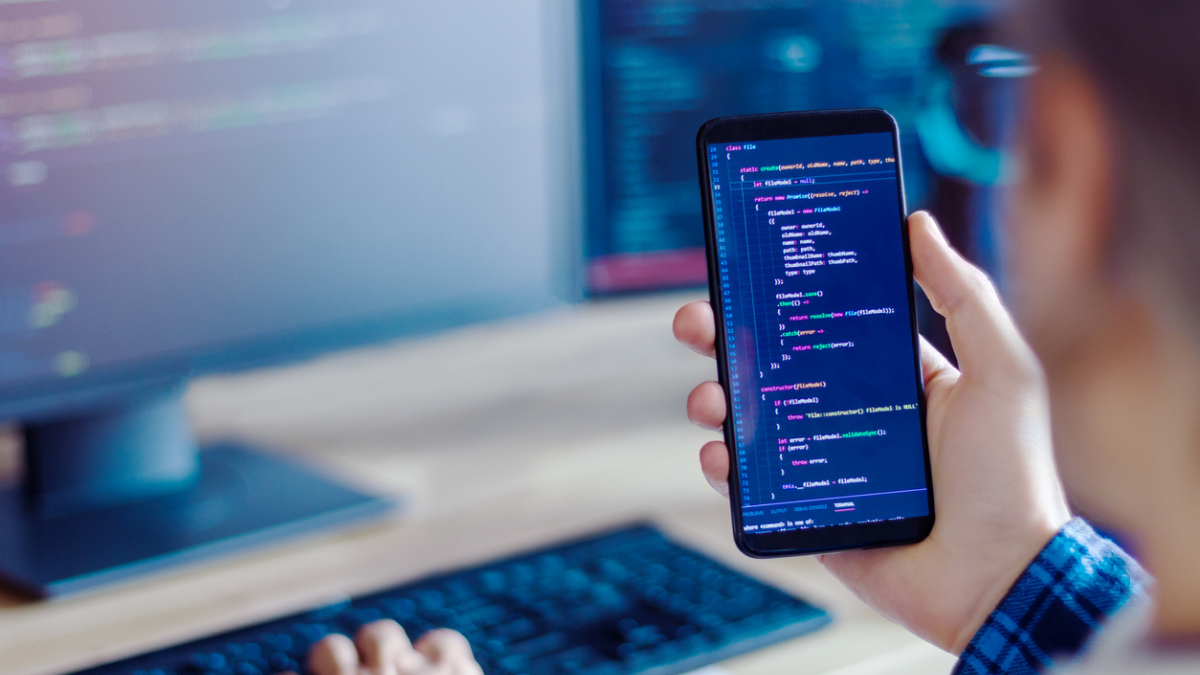
If you have experimented with making an app, chances are you have probably used Fragments. But have you heard about the Fragment LifeCycle?
It looks something like this :

If you are thinking “ That looks like the Activity LifeCycle! ”, then yes you are right. Fragments have similar lifecycles to Activities. What’s the difference then? Well, a major one would be :
In activities we use the onCreate() Method to inflate the layout and bind views while in case of fragments the layout is inflated in onCreateView() Method and we bind views in the onViewCreated() method.
Now that we know what the LifeCycle looks like, let’s understand it. So open your fragment and override the above methods like this :
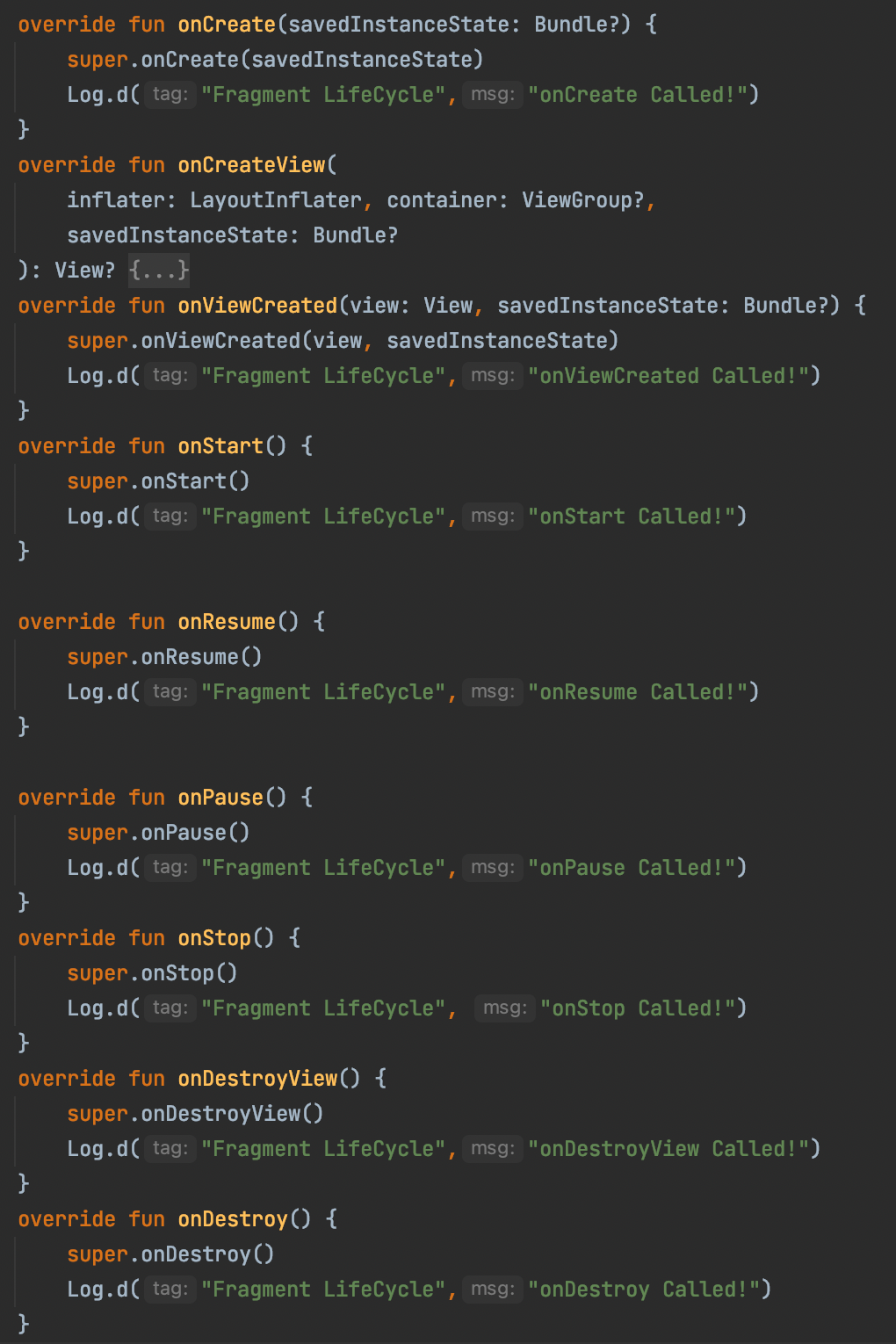
When you open your fragment you will notice these in your Logcat :
Fragment LifeCycle: onCreateView Called!
Fragment LifeCycle: onViewCreated Called!
Fragment LifeCycle: onStart Called!
Fragment LifeCycle: onResume Called!
Now if you minimize your app you will notice :
Fragment LifeCycle: onPause Called!
Fragment LifeCycle: onStop Called!
And if you open it again you will notice :
Fragment LifeCycle: onStart Called!
Fragment LifeCycle: onResume Called!
That makes things a little clearer, but are onPause() and onResume() only called with other methods? No, onPause() is called when the app loses focus and OnResume() is called when it is in focus. To test this just add a button to your fragment layout and on its Click Listener write the following code :
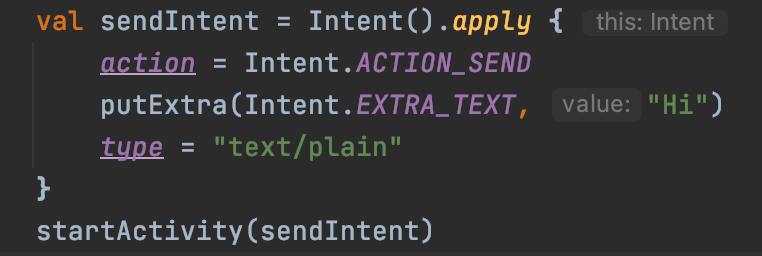
This is just an implicit intent to send some data to someone else. When you click this button you will notice in your Logcat :
Fragment LifeCycle: onPause Called!
Now if you click outside the dialogue you will notice :
Fragment LifeCycle: onResume Called!
But what happens to the ViewModel during all this? Well let’s experiment with that as well. Add the following methods in your ViewModel :

Now when you start your app you will also notice :
Fragment LifeCycle: viewModel Created!
Now rotate your mobile or emulator, you will not see the “viewModel Destroyed” entry in your Logcat, this is because the ViewModel does not get destroyed on configuration changes and thus can be used to store data.
But does the ViewModel get destroyed if we navigate to another fragment and what about the fragment itself? Let’s try it out!
Add another button to your layout and in its ClickListener add the following code :

Note : You will need to setup Navigation Component to navigate between fragments.
You will notice the following in your Logcat :
Fragment LifeCycle: onPause Called!
Fragment LifeCycle: onStop Called!
Fragment LifeCycle: onDestroyView Called!
Note : If you add Debug statements to Fragment2’s LifeCycle methods, you will notice that Fragment2’s onCreate(), onCreateView(), onViewCreated(), onStart() and onResume() methods are called before the above appears in the Logcat.
As you can see the ViewModel still does not get Destroyed and if you navigate back to the first fragment using the back button then you will see that any data stored in the ViewModel is preserved and the onCreate() method is not called as the fragment was never destroyed.
When does the ViewModel get destroyed? Close the app and you will notice in the Logcat :
Fragment LifeCycle: onPause Called!
Fragment LifeCycle: onStop Called!
Fragment LifeCycle: onDestroyView Called!
Fragment LifeCycle: viewModel Destroyed!
Fragment LifeCycle: onDestroy Called!