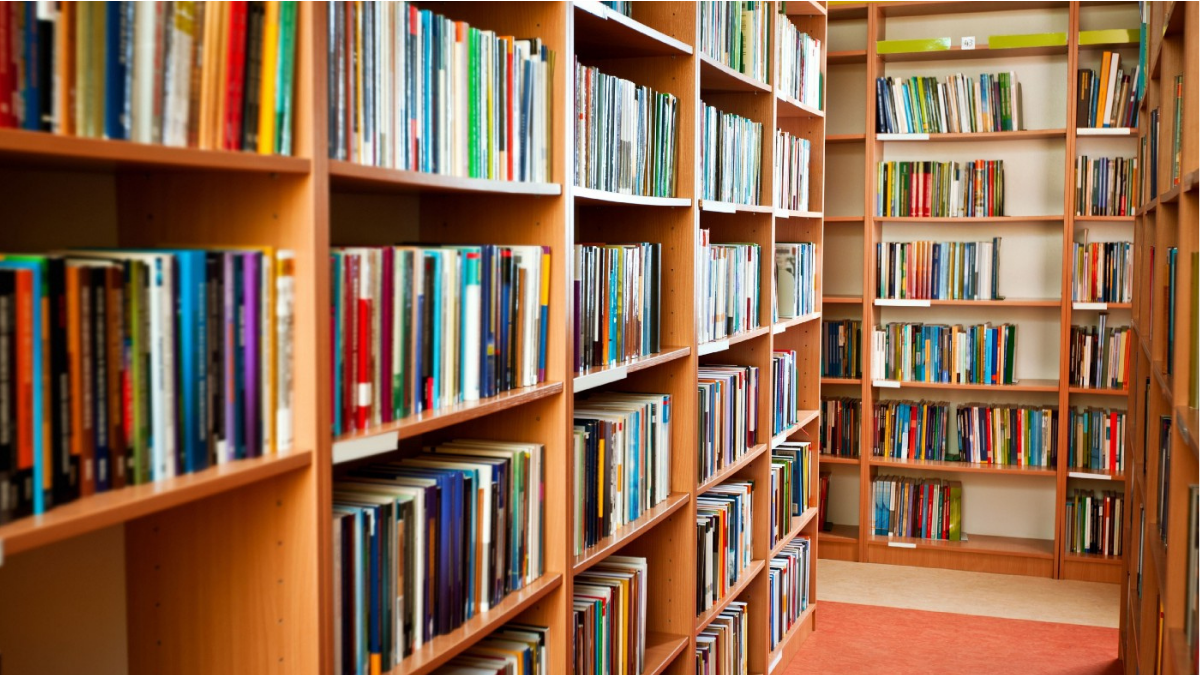
I always wonder how android dependency works.
An Android library is structurally the same as an Android app module. It can include everything needed to build an app, including source code, resource files, and an Android manifest. However, instead of compiling into an APK that runs on a device, an Android library compiles into an Android Archive (AAR) file that you can use as a dependency for an Android app module.
In this tutorial, we are going to create an Android Library, and we also see how we can distribute it to JitPack so we can use it as a dependency Gradle in other Android projects.
So let’s start
Steps which we are following
We are creating a simple toast library
- Step 1: Create a new Android app that showcases the library
- Step 2: Create a new library module within the Android app
- Step 3: Write the code for your custom library
- Step 4: Add the library as a dependency to the project
- Step 5: Publish your library to Github
- Step 6: Setup to share your library with any other android project using
Jitpack
Step 1: Create a new Android app that showcases the library
Open Android Studio and create a new project and name it as ToasterExample

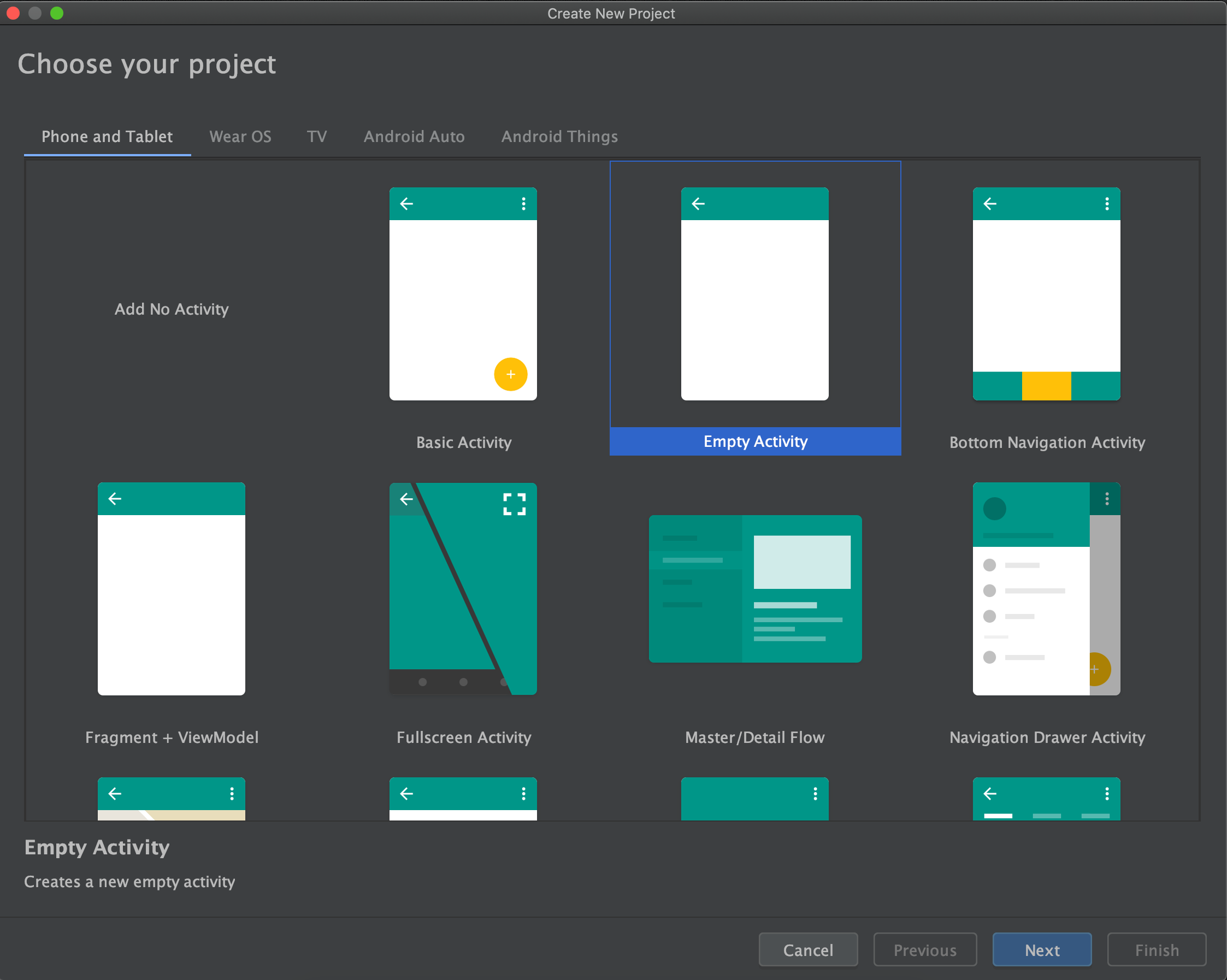
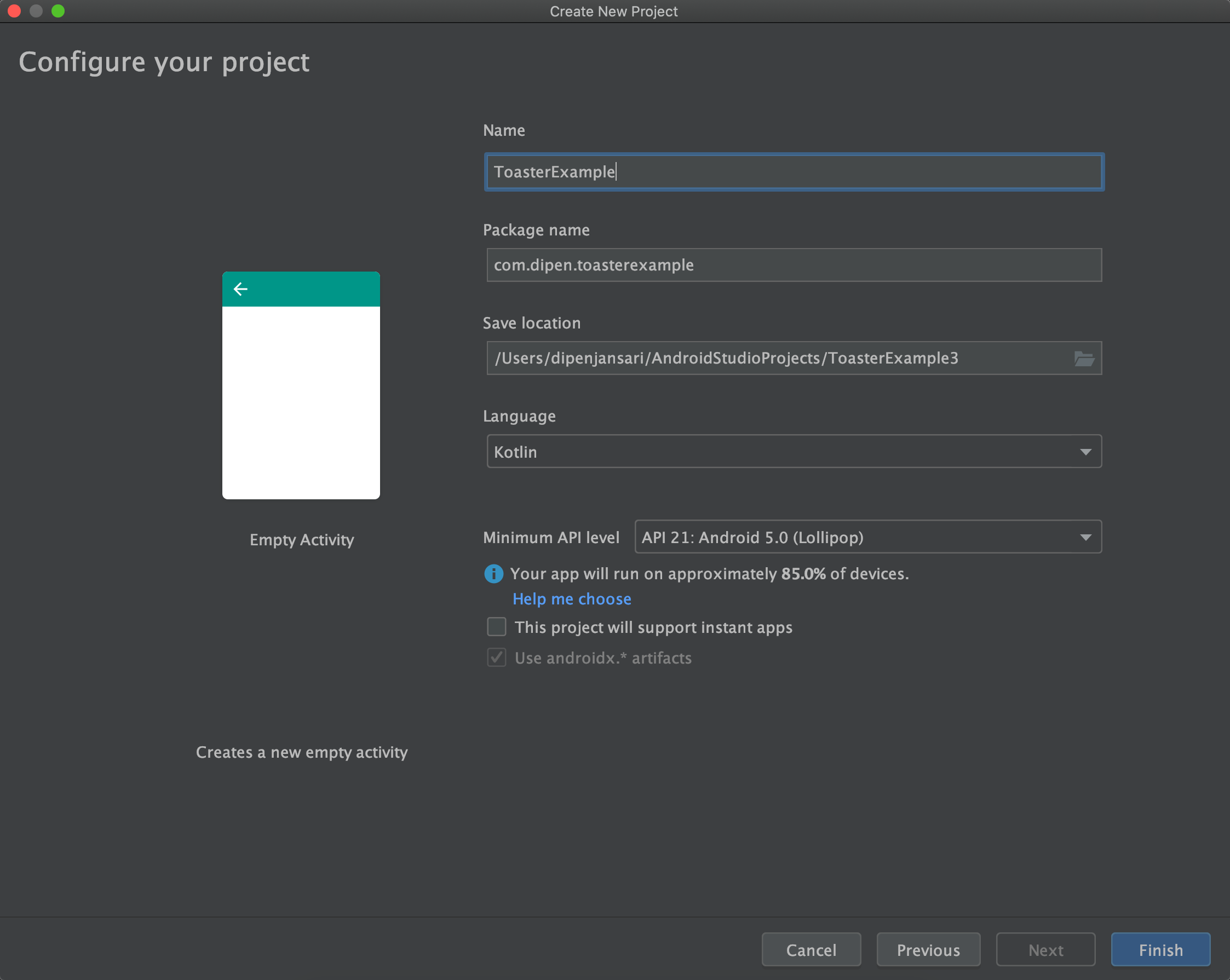
Click on Finish and your project will be ready.
Step 2: Create a new library module within the Android app
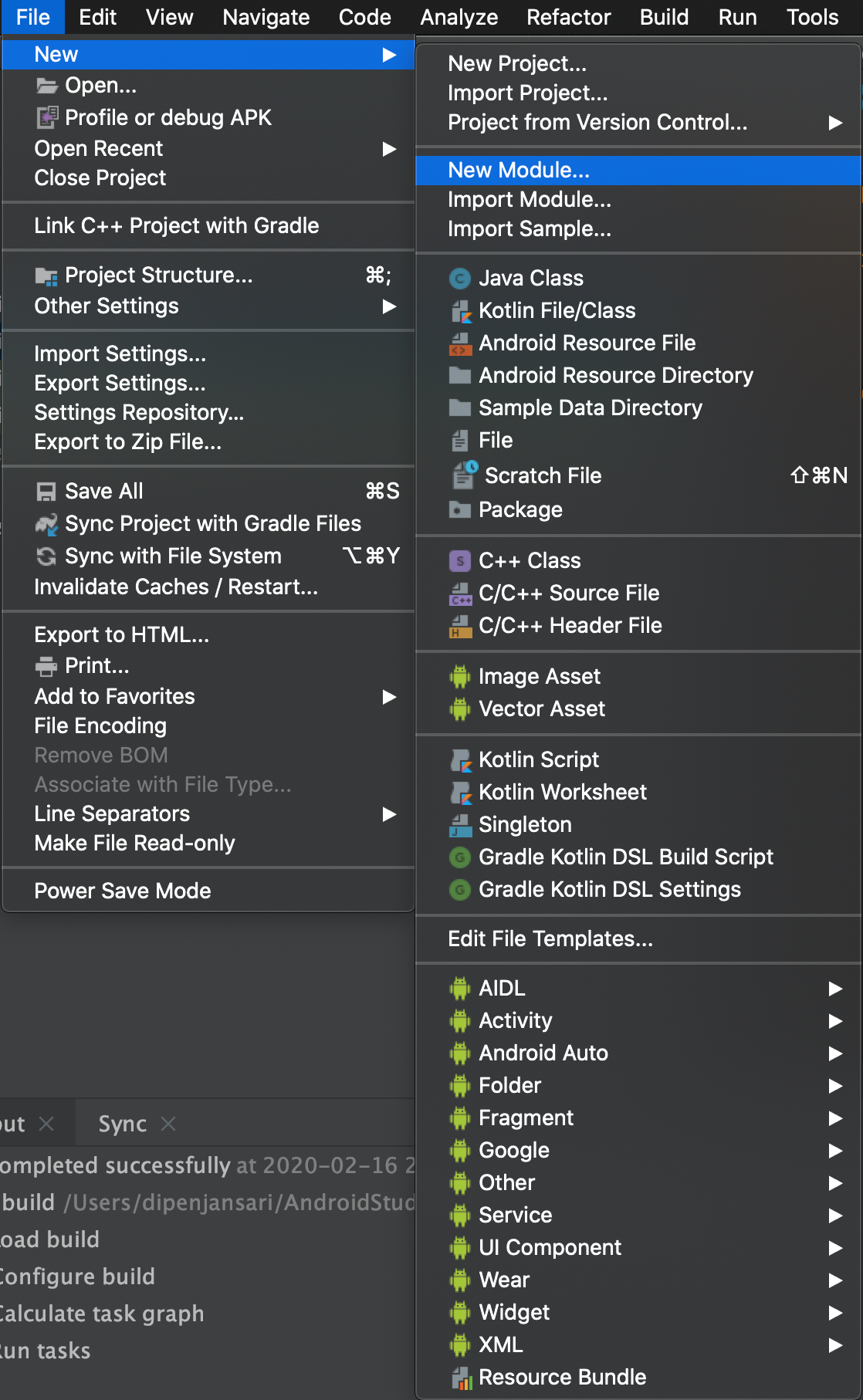
Select Android Library from the options and click on Next and Finish it
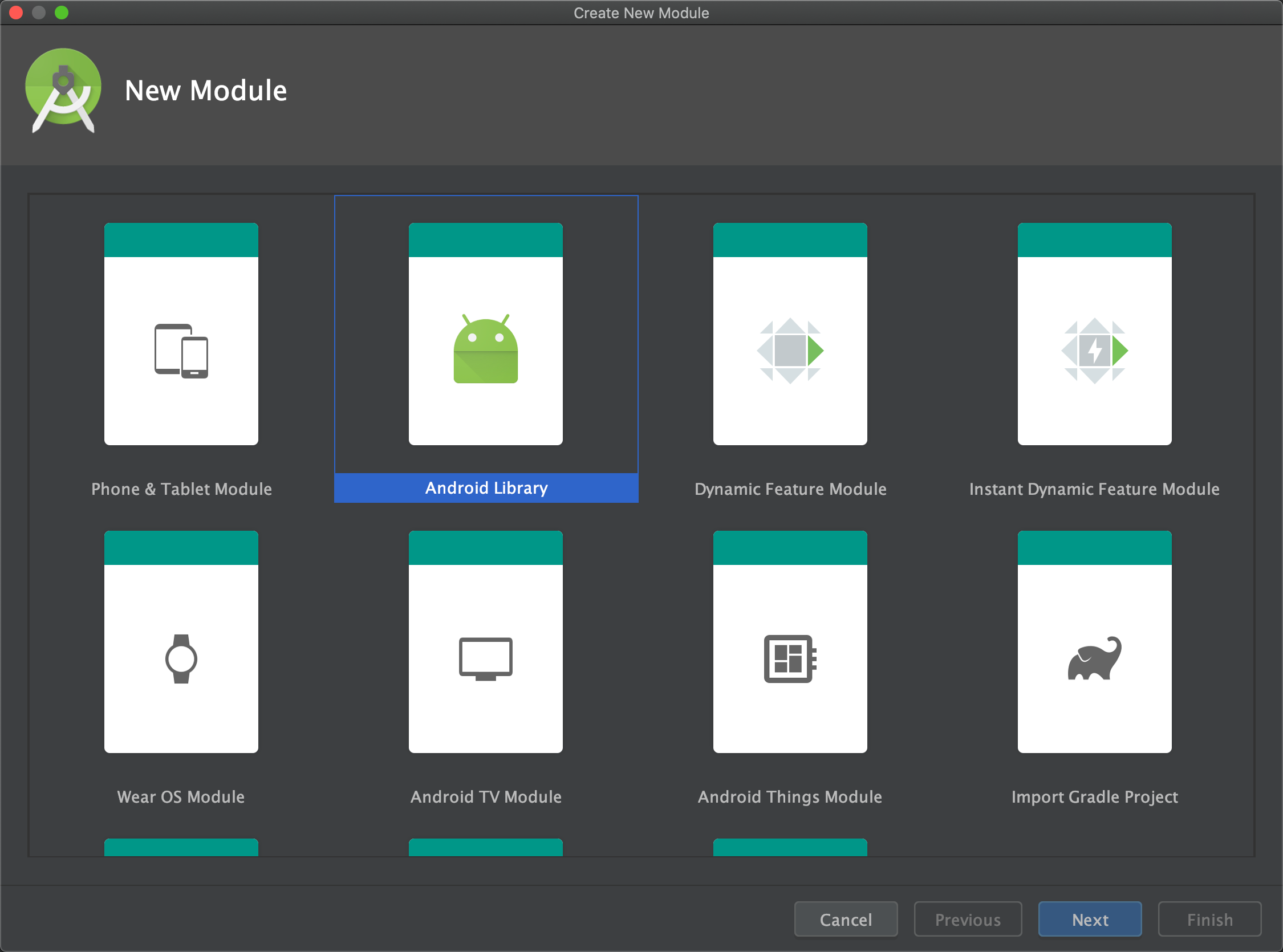

Step 3: Write the code for your custom library
- Now we need to add code in our library. Create a new class in your module and name that class ToasterMessage


Modify ToasterMessage.kt as follows
class ToasterMessage {
companion object {
fun toastMessage(context: Context, message: String) {
Toast.makeText(context, message, Toast.LENGTH_SHORT).show()
}
}
}
Step 4: Add the library as a dependency to the project
Open your app’s build.gradle file and add the following inside dependencies
after // Testing
dependencies
// added validatetor Android library module as a dependency
implementation project(':toasterlibrary')
Now sync up your project Gradle files. That is it. You have just added the toasterlibrary Android library module to your app.
This is one of the ways of adding an Android library to an app project. There are more ways which will be discussed later on in the tutorial.
Now that you have the toasterlibrary library added as a dependency, you can reference the library code in your app.
Note: you will be referencing the Java-based Android library inside the Kotlin MainActivity class. There is no difference in usage except for following the Kotlin syntax
So our Library is completed and now its time to publish the library. To publish the library all you need is a GitHub repository.
Step 5: Publish your library to Github
There are several ways available to publish a project to Github
We will share our project to GitHub via Android Studio only


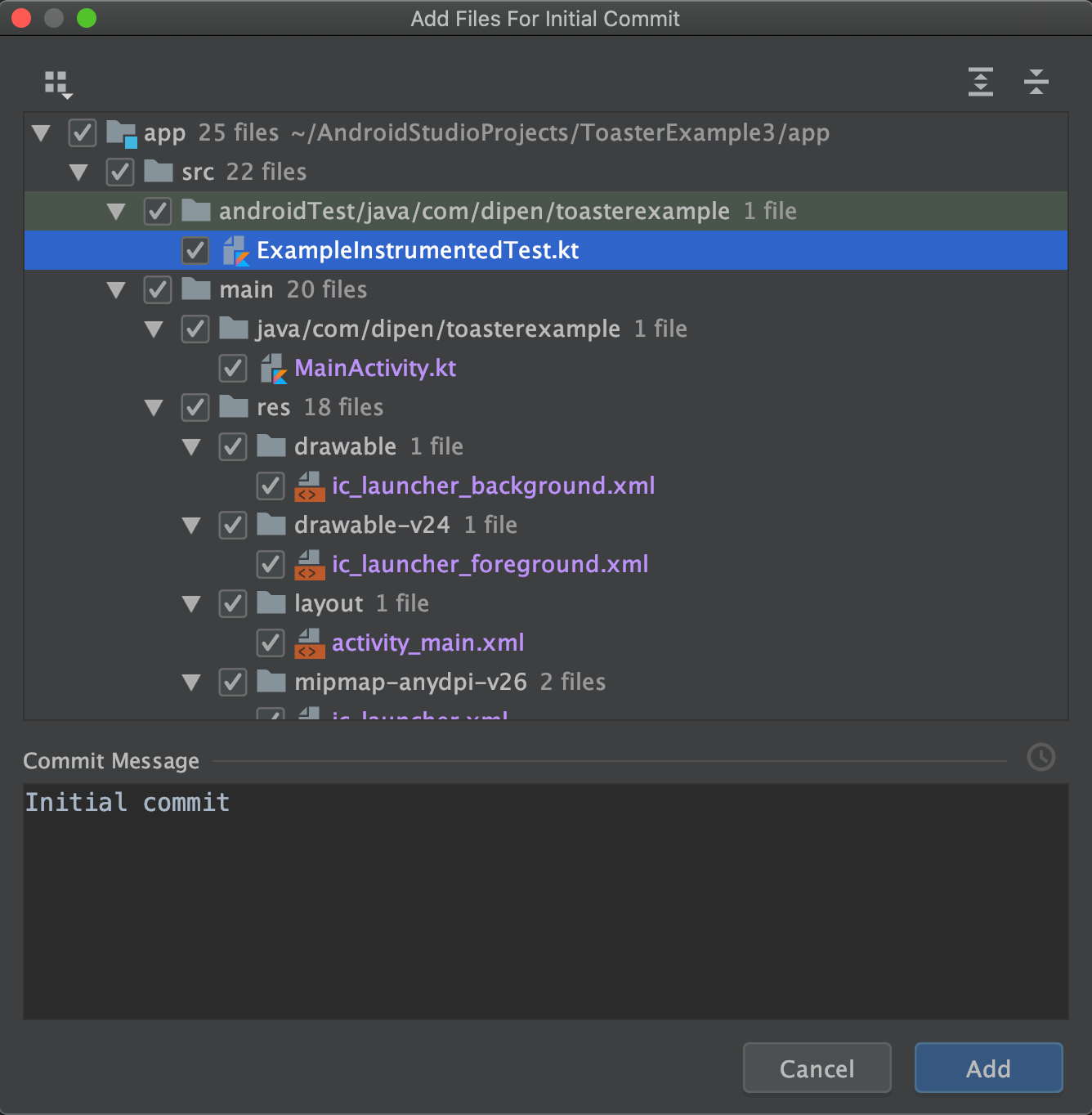
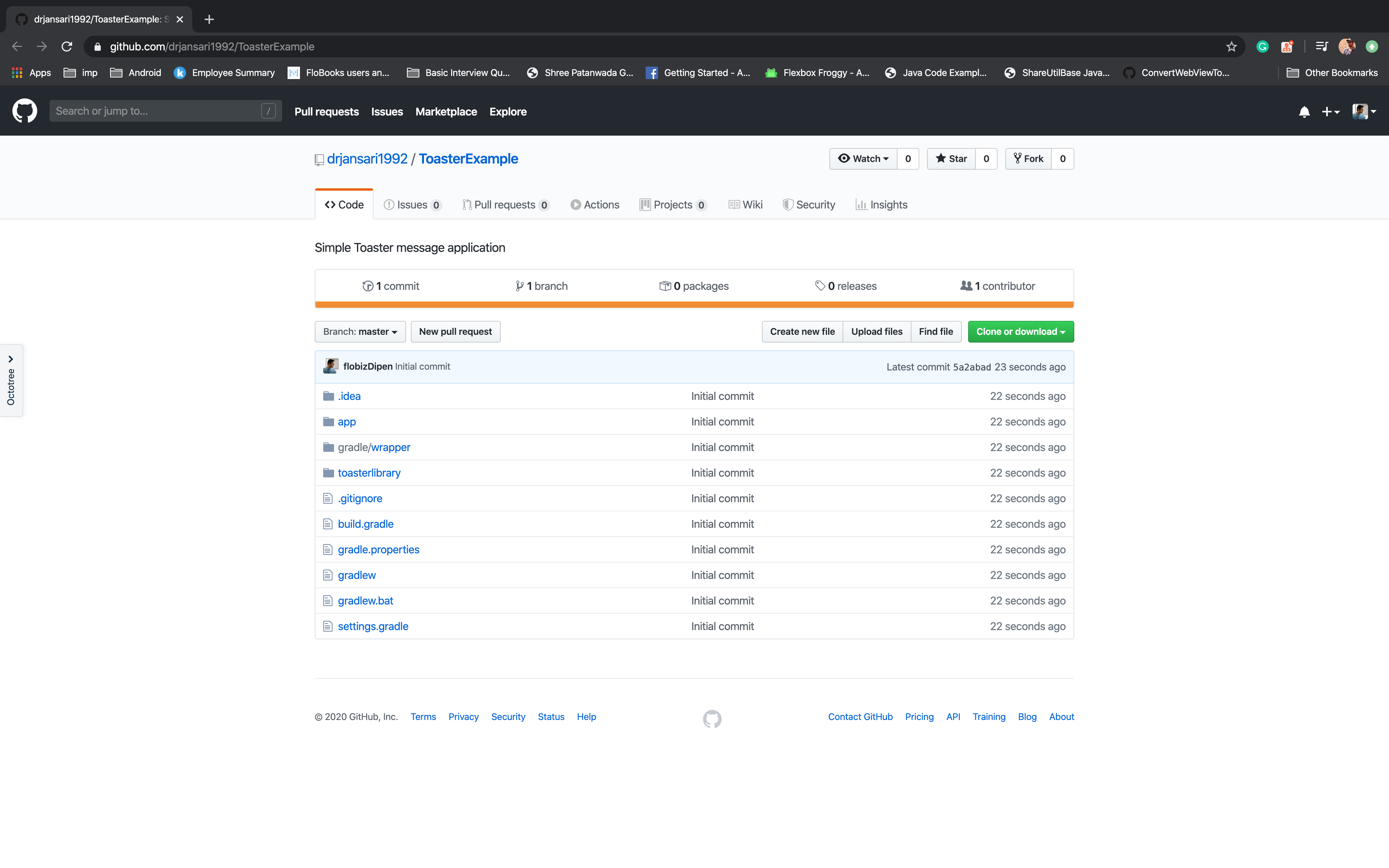
Now in GitHub repo click on Release and create a New Release


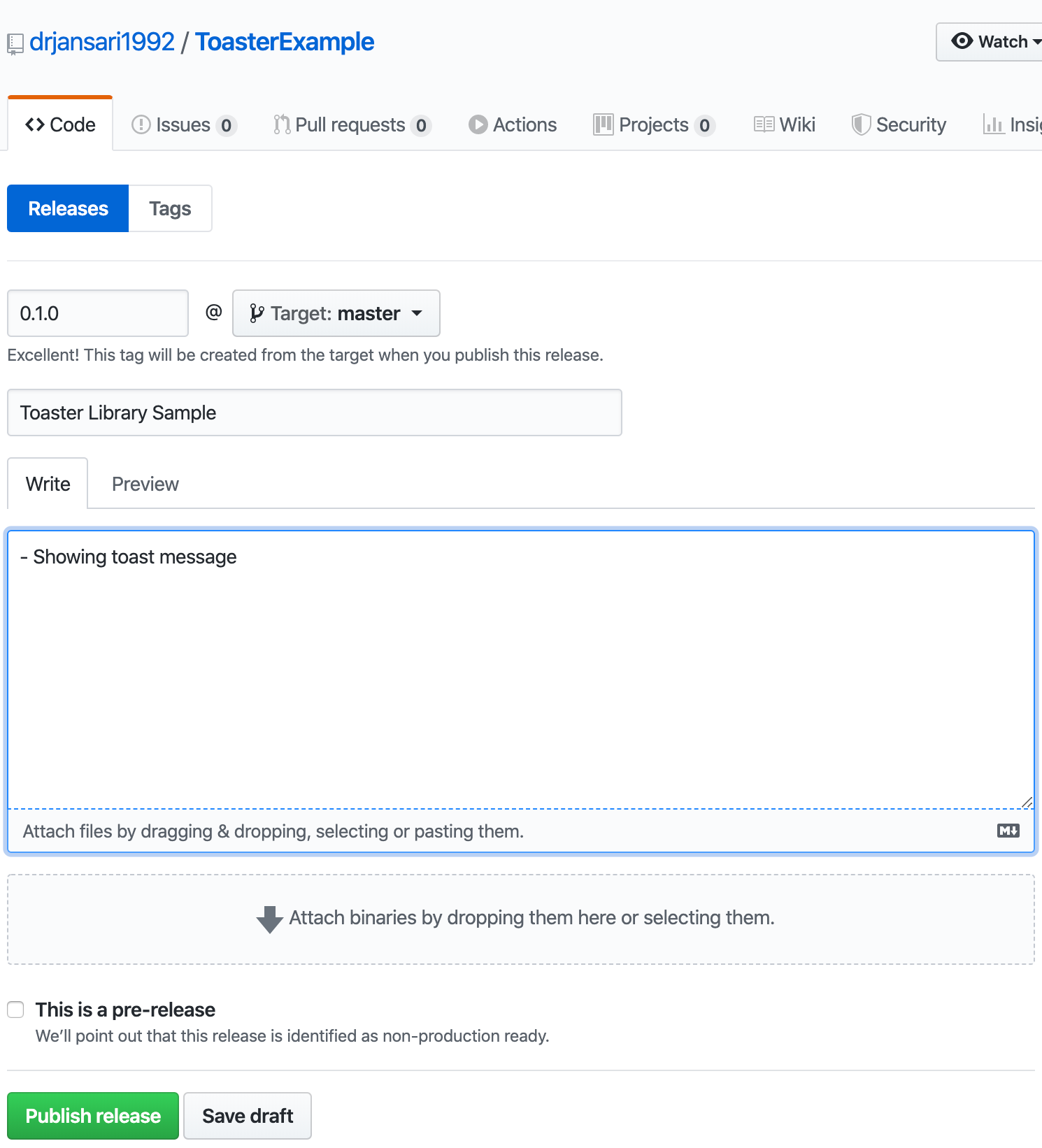
Publish the release.
Step 5: Publishing your Android library
You have 3 options here
For this tutorial, we are going to use Jitpack to publish our Android Library.
Insert your repository address and click on Lookup. Your releases will be listed. Now click on Get it.

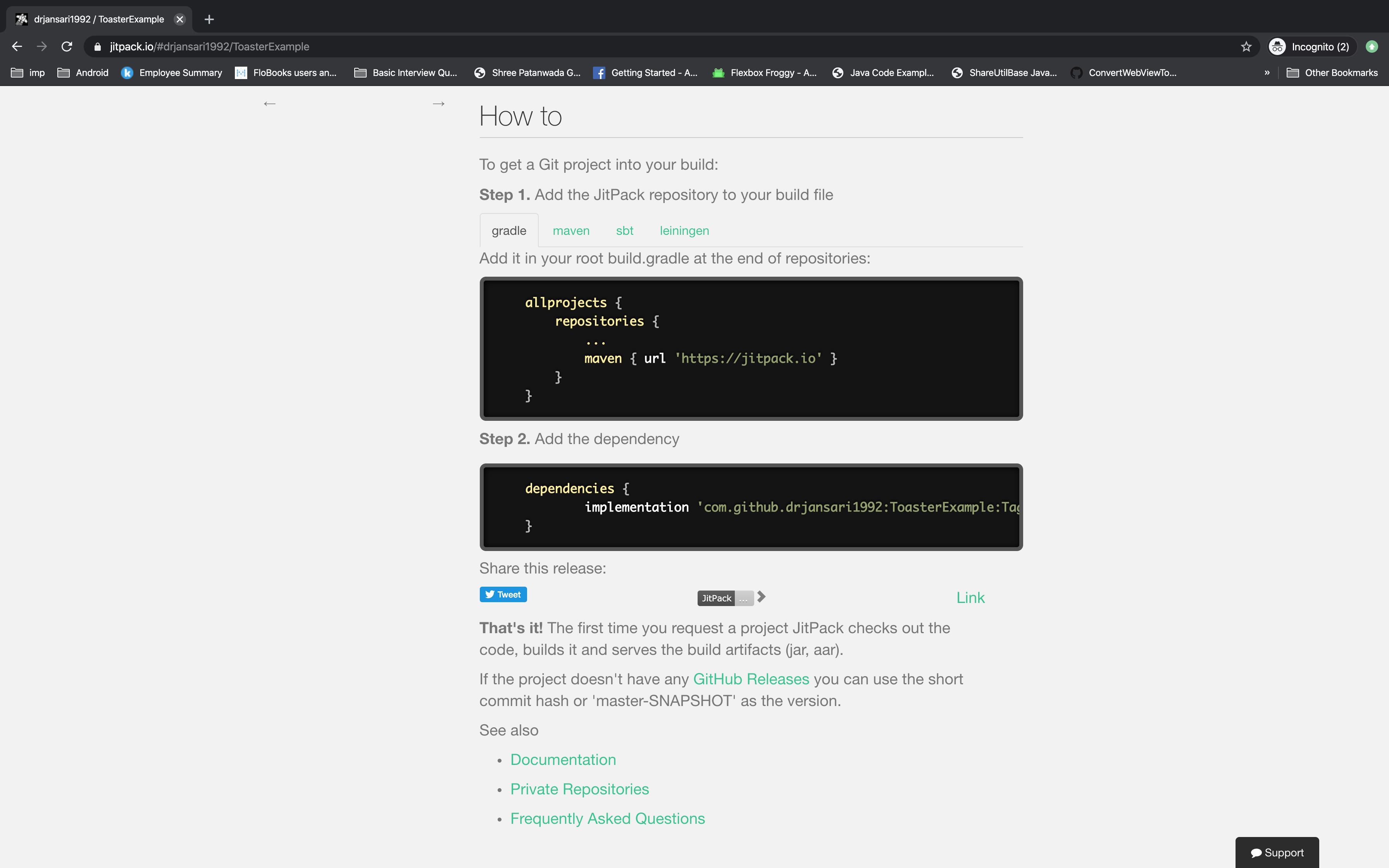
Kudos, your Android Library is published and it is ready to use
Note: Above example is for the public repo, if you have private rapo than visit this link https://jitpack.io/private#auth
Using the Android Library
Now you can use this android library in any of your projects.
In your project’s build.gradle add the following line
maven { url ‘https://jitpack.io’ }
and in your app’s build.gradle add the dependency
compile ‘com.github.aj019:Toaster-Library:0.1.0’
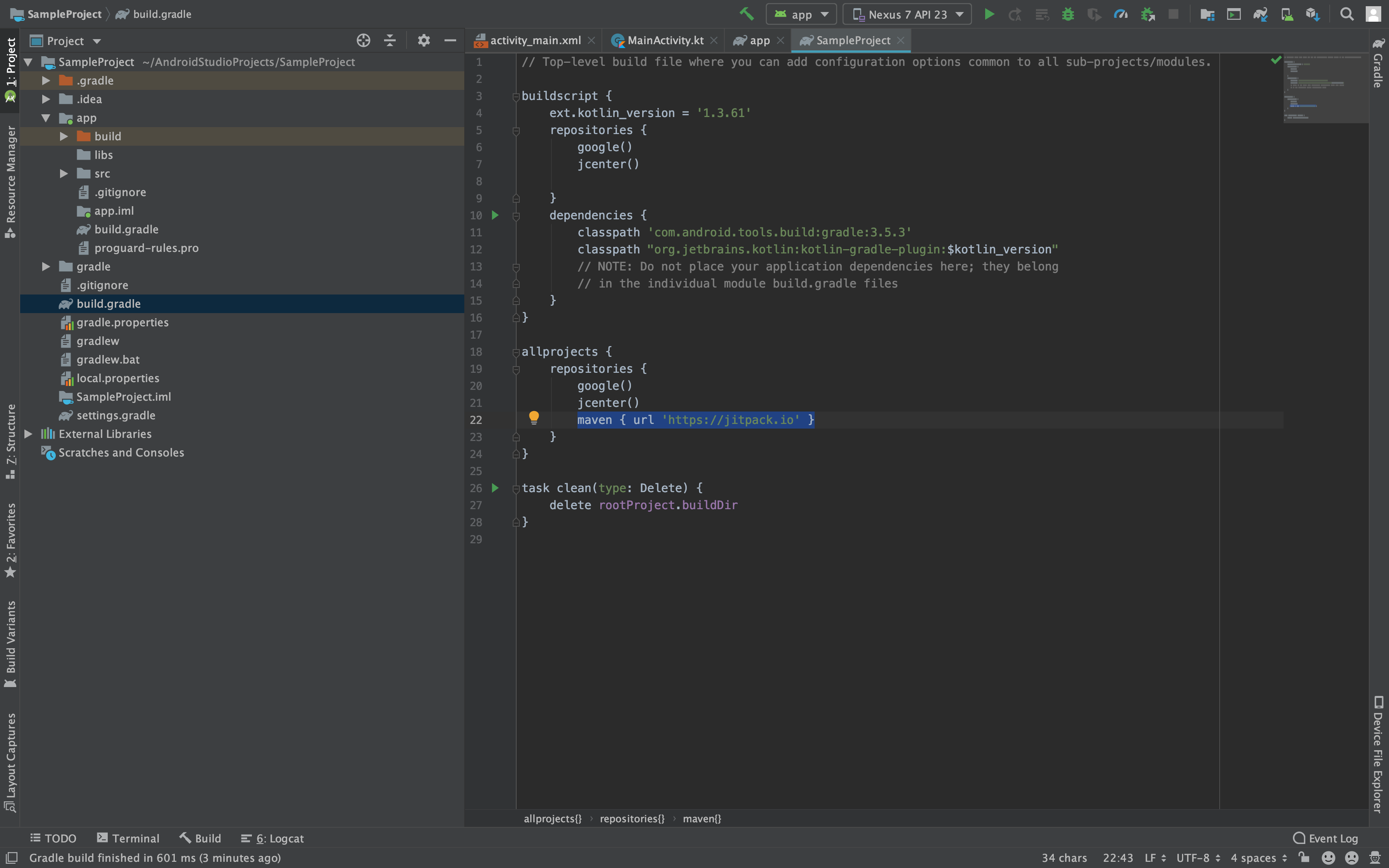

Thanks for reading this article
Hopefully, after finishing this tutorial you have a solid understanding of building and publishing better Android libraries.
If you want to learn more about Android library development, check out Google’s official documentation.
If you have any questions or comments, please join the forum discussion below!
Source Code is available on GitHub at:
https://github.com/drjansari1992/ToasterExample
Stay connected on LinkedIn Twitter and StackOverFlow.
Be sure to clap/recommend as much as you can and also share it with your friends.